Wengier
系统支持
             “新DOS时代”站长
积分 27734
发帖 10521
注册 2002-10-9
状态 离线
|
『楼 主』:
DJGPP/GCC介绍
An Introduction to GCC
1. Using GCC in the lab
1.1 Creating a simple C program
1.1.1 Editing
1.1.2 Compiling
1.1.3 What do all those commands mean?
1.1.3.1 gcc filename
1.1.3.1.2 -Wall
1.1.3.1.3 -O
1.1.3.1.4 -o hello.out
1.1.3.2 go32 hello.out
1.1.3.3 coff2exe hello.out
1.1.4 Error Messages
1.2 More Complex C Programs
2. Using GCC at home
2.1 Obtaining GCC
2.1.1 In the lab
2.1.2 On the Web
2.2 Installation
A. Useful C Links
Conventions
Text in this font should be entered as written, and followed by pressing the ENTER key. For example:
Type this sentence.
means you should type "Type this sentence." (without the quotes), and then press the ENTER key. If you see {TAB} it means press the TAB key.
1. Using GCC in the lab
GCC is a compiler which translates a program written in C (which humans can read) to machine code which the computer can "understand", and execute. The Watfor77 program that was used in a previous portion of the course is a combined compiler/editor. The difference is that with Watfor you could start "watfor77" write a program and save and run it within the same program. GCC is not like this. GCC is only a compiler. It translates already existing files. The importance of this will be demonstrated through examples.
1.1 Creating a simple C program
1.1.1 Editing
The first step in creating a program with GCC doesn't involve GCC at all. You will have to enter a program and save it before you use GCC. A typical program used for entering a text file (which is all a C program is before its compiled) is notepad. To start notepad, use Start|Programs|Accessories|Notepad. In notepad enter the following program:
#include
int main(int argc, char *argv[])
{
printf("Hello World.\n" ;
return 0;
}
Save the program as "a:\hello.c".
1.1.2 Compiling
Now you start using GCC. Start an MS-DOS session, either by using Start|Programs|MS-DOS or by double-clicking on the MS-DOS icon on the desktop. Then type:
e:\gcc\gccsetup
This sets things up so the computer can find GCC and related programs. (NB: Running gccsetup by pointing and clicking won't do what you want; it would only set things up for the brief period it was running). You need to use the gccsetup command each time you open a new DOS window (and want to use GCC).
Then type:
gcc -Wall -O -o hello.out hello.c
It will work away with no apparent action for a while, then you will see the DOS prompt again (assuming no errors in entering the program or command). If you do a "dir" you will see that a new file has been created called "HELLO.OUT". To run the program you can type:
go32 hello.out
You should see two lines of output, the second being "Hello World!"
If you want the program to be executable without using go32 (e.g. by just typing "HELLO" , you would type:
coff2exe hello.out
If you do a "dir" you should now see another file called "HELLO.EXE". This can be run just like any other DOS program (e.g. by typing
hello
1.1.3 What do all those commands mean?
1.1.3.1 gcc filename
"gcc filename" is the command you get if you ignore all "options" (preceded by -). In the above example this would be "gcc hello.c". Gcc is the name of the compiler program, and filename is the C source file to be compiled (translated).
1.1.3.1.2 -Wall
This option tells the compiler to tell you almost everything that it can detect that you might have done wrong (i.e. it will issue a message warning you about parts of you program that look like they could be in error, but aren't definite errors).
1.1.3.1.3 -O
This option stands for optimize. It tells the compiler to try to write as quick and compact code as possible. This isn't necessary for the size of programs you will be writing, but it is necessary for some warnings (see -Wall) to be discovered.
1.1.3.1.4 -o hello.out
This option tells the compiler to name the output file hello.out. If you don't have a "-o filename" option the output file will named a.out.
1.1.3.2 go32 hello.out
"go32" is a program that allows GCC programs to be run without fully converting them to a DOS executable format. It and "coff2exe" are required under DOS because GCC was written for UNIX and it's output is geared to that platform. "go32" is a program that allows programs not yet converted to DOS format to be run under DOS. (See also coff2exe)
1.1.3.3 coff2exe hello.out
"coff2exe" is a program that converts GCC output programs into DOS executables (i.e. program that run under DOS without an additional program required). This is necessary because GCC was written for UNIX and standard UNIX program formats are not recognized by DOS. The end result is that an extra step is required to get GCC programs to work compared to GCC under UNIX, or native DOS compilers.
"coff2exe" takes a file and create an equivalent file named "name.exe" (NB: If the original file had an extension such as .OUT it is replaced by .EXE, so HELLO.OUT becomes HELLO.EXE - this is due to a limitation in DOS).
1.1.4 Error Messages
If there are errors in your program that GCC can detect it will produce error messages. Often there will be too many messages for a single screen. In this case its a good idea to type the standard gcc command (e.g. "gcc -Wall -O -o hello.out hello.c" followed by ">errors.txt". For example you could type:
gcc -Wall -O -o foobar.out foobar.c >errors.txt
If you were compiling a program named "foobar.c" and wanted your errors saved to "errors.txt". You can then view errors.txt using any standard editor (such as notepad).
Some important notes on error messages
Don't trust compiler line numbers: The compiler often will fail to notice a mistake on the line which originally caused it, so you should look at lines above the line with the error as well.
Don't trust errors after the first few. You could say that once the compiler gets confused it has a hard time getting over it. This means that if you see huge numbers of errors the first time you compile, you don't have to panic right away - many of the errors will probably disappear once you fix the first one or two offenders
1.2 More Complex C Programs
1.2.1 Assignment 1a in C
/* include the standard C libraries so your functions will work */
#include
#include
#include
/* This is the 'main' function. This is where program execution begins */
void main(void)
{
/* Declare variables */
int operator;
float value;
float newvalue;
/* loop forever (unless 'q' or 'Q' entered) */
while(1) {
/* ask for input */
printf("Please input operation and value\n" ;
printf("Where operation is an integer,\n" ;
printf("1 - Convert from Centigrade to Fahrenheit\n" ;
printf("2 - Convert from Fahrenheit to Centrigrad\n" ;
/* Read input */
scanf("%d%f", %operator, %value);
/* Exit program? */
if (operator == 0)
{
break; /* exit loop */
}
/* number in Fahrenheit */
if (operator == 1)
{
newvalue = (value - 32) * ((float)5 / 9);
printf("%f in Centigrade is %f in Fahrenheit\n.", value, newvalue);
continue;
/* go back to beginning of loop (while) */
}
if (operator == 2)
{
newvalue = ((value) * ((float)9 / 5) + 32);
printf("%.2f in Fahrenheit is %f in Centigrade\n", value, newvalue);
continue; /* go back to beginning of loop (while) */
}
}
}
2. Using GCC at home
"Ok, I've been using GCC in the lab and come to know and love it - now how to I take it home?" Assuming you have at least a 386 you can use the instructions below.
2.1 Obtaining GCC
2.1.1 In the lab
The first step to running GCC on your home computer is to get a copy of it. The easiest way to do this is take six floppies (3.5" disks) and copy the contents of E:\course\cs150\GCCDISKS\DISK1 through E:\course\cs150\GCCDISKS\DISK6 to A:, labelling each disk appropriately.
Detailed instructions follow.
1) Make sure you have six blank formatted disks
2) Login to a lab computer
3) Start an MS-DOS prompt (as you would to start Watfor77, except don't enter watfor77
4) Place a disk labelled "GCC Disk 1" in drive A:
5) Type:
E:
CD \course\cs150\GCCDISKS
COPY DISK1\*.* A:
6) Repeat the steps 4 and 5, replacing "DISK1" with "DISK2" through "DISK6".
Disks 1-5 should now contain a file named "GCC.ZIP". Disk 6 should have some additional files.
2.1.2 On the Web
This is recommended for experienced users only!
IMPORTANT: If you choose to use a copy of GCC obtained from the Web it is your responsibility to make sure that the code you write will compile and execute correctly on the machines in the lab. Be warned: The web version is different and may fix some bugs and/or introduce new bugs/features into GCC that aren't in the version on the lab computers, but the TA's will be marking based on the lab computer installation.
Two sites for information/downloading are:
A helpful site for information: http://www.cs.mun.ca/~cfollett/djgpp/indexold.html
The official distribution site: http://www.delorie.com/djgpp/
2.2 Installation
Now that you've got GCC on disk you can install it on the computer of your choice.
1) If you aren't installing from drive a: to c:\gcc, edit install.bat and gccinst.bat files to use the appropriate drives/directories. (if you're not sure you should just use the files as they are)
e.g. The following are the default files.
install.bat
echo off
copy gccinst.bat c:\
copy pkunzip.exe c:\
c:
\gccinst.bat
gccinst.bat
echo off
c:
mkdir gcc
cd gcc
c:\pkunzip -d a:\gcc.zip c:\gcc
del c:\pkunzip.exe
mkdir c:\tmp
and the following files are modified to use b: and d:\apps\gcc, and use c:\temp as the directory used for temporary files
install.bat
echo off
copy gccinst.bat d:\
copy pkunzip.exe d:\
d:
\gccinst.bat
gccinst.bat
echo off
d:
mkdir \apps\gcc
cd \apps\gcc
d:\pkunzip -d b:\gcc.zip d:\apps\gcc
del d:\pkunzip.exe
mkdir c:\temp
2) Put Disk 6 in drive a: (unless you've modified the install BATch files to use another drive, as explained above).
3) At the DOS prompt type:
a:\install
You should put each disk in the drive as requested.
4) If you modified install.bat/gccinst.bat you will also need to edit gccsetup.bat to use the correct drive and directory (gccsetup.bat can be found in the drive:\directory you used to replace c:\gcc).
original gccsetup.bat (note that the /'s are correct)
mkdir c:\tmp
set path=c:\gcc\bin;%path%
set DJGPP=c:/gcc/djgpp.env
set GO32=emu c:/gcc/bin/emu387
modified as in example for install.bat/gccinst.bat
mkdir c:\temp
set path=d:\apps\gcc\bin;%path%
set DJGPP=d:/apps/gcc/djgpp.env
set GO32=emu d:/apps/gcc/bin/emu387
note that if you have a 486DX or better (or have 387 math coprocessor) you can put "rem " in front of "set GO32=..." - as with most things for this installation, if you don't know, leave it alone.
5) If you modified c:\tmp to some other drive you will need to modify djgpp.env as well.
(djgpp.env can be found in the same place as gccsetup.bat). The only part you should need to modify is:
+USER=dosuser
+TMPDIR=c:/tmp
+GO32TMP=c:/tmp
If you decided to use c:\temp as the temporary file directory as in the examples above, you would use (leaving the rest of the file unchanged):
+USER=dosuser
+TMPDIR=c:/temp
+GO32TMP=c:/temp
6) At this point you should be able to use GCC as you would in the lab (that is: You need to run gccsetup.bat (located in c:\gcc) each time you start a new DOS session (i.e. open an MS-DOS window) if you want to use GCC. (See Section 2).
7) If you want to be able to use GCC without running gccsetup each time you can follow the instructions below. (It is assumed you are fairly computer literate for the instructions for these procedures).
Add the following to autoexec.nt (autoexec.95 for Win95 ?), assuming a default drive/directory setup. (If you're using Win 3.1 you would edit autoexec.bat)
PATH=c:\gcc\bin;%PATH%
SET DJGPP=c:/gcc/djgpp.env
8) To test the installation, at the DOS prompt (don't forget gccsetup), type:
c:
cd \gcc\samples\hello
testit
You should see a message, a request to press a key, then when you press a key messages should scroll by, and finally you should see, "Hello! This is the test program."
9) To further test the installation, start a new DOS session (remembering gccsetup), and type:
c:
cd \gcc\samples\hello
tryit
You should see messages scroll by, and "Hello! This is the test program."
10) GCC is now installed successfully!
|

Wengier - 新DOS时代
欢迎大家来到我的“新DOS时代”网站,里面有各类DOS软件和资料,地址:
http://wendos.mycool.net/
E-Mail & MSN: wengierwu AT hotmail.com (最近比较忙,有事请联系DOSroot和雨露,谢谢!)
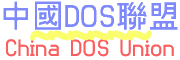 |
|